|
Programs
File Backupper
Digitize Plot To Data
Ready Replace
Scrabbler
Tutorials
Comsol 4.2 Tutorials
Gwyddion Tutorials
VB 6.0 Tutorials
Tutorials: else
|
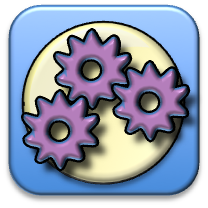 |
Tutorials |
VB 6.0 Tutorial: Dialog without OCX 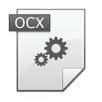
Here is a tutorial for how to use the common open/ save dialog box from the comdlg32.ocx without using the ocx file and without having to register it and without having administrator rights.
If you use the comdlg32.ocx in your program and want to give the compiled program to someone, you have to deliver the ocx file and register it, since it is often not installed on some Windows systems such as Windows 7.
Problem
This is the error message you would get when you try to open a program using this ocx file if you wouldn't register it or if you do not have the ocx file on your computer:
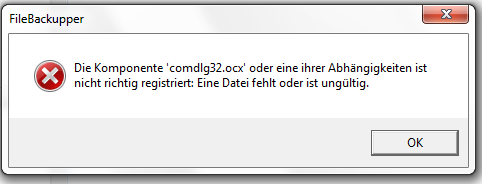
Error message in German:
Die Komponente 'comdlg32.ocx' oder eine ihrer Abhängigkeiten ist nicht richtig registriert. Eine Datei fehlt oder ist ungültig.
Error message in English:
Component 'comdlg32.ocx' or one of its dependencies not correctly registered: a file is missing or invalid.
The usual way is to copy the ocx file to the C:\Windows\system32 folder (in Windows XP) or the C:\Windows\SysWOW64\ folder (in 64bit Windows Vista/ Windows 7). After that you have to register it using the command promt (go to Start -> Run -> and type in "cmd"). In there you just type
following text or download and execute the bat file:
Windows 95, 98, or Me:
C:\Windows\system\comdlg32.OCX
regsvr32 comdlg32.ocx
|
 |
In Windows NT, 2000:
copy comdlg32.ocx
C:\WINNT\system32\comdlg32.OCX
regsvr32 comdlg32.ocxpan> |
 |
In Windows XP:
copy comdlg32.ocx
C:\Windows\system32\comdlg32.OCX
regsvr32 comdlg32.ocx |
 |
In Windows 7:
copy comdlg32.ocx C:\Windows\SysWOW64\comdlg32.ocx regsvr32 C:\Windows\SysWOW64\comdlg32.ocx |
 |
Klick on the icon
to download the BAT file with this commands.
Writing a file to the system folder (e.g. into C:\Windows\SysWOW64\) on Windows 7 and registering this ocx module however requires administrator rights. If you don't have them, you will get an error message with following number: 0x8002801c
Solution
In the following project you will learn how to directly use the comdlg32.dll with APIs on your system which is already installed in Windows 7 in contrast to the comdlg32.ocx file.
You thus don't need to register or deliver the comdlg32.ocx file with your program and you don't need administrator rights to open your program.
Keywords:
comdlg32.ocx common dialog replacement
common dialog with Win32 API
Download VB6.0 Project with solution
The source code for this project:
Option Explicit
'command button for open dialog:
Private Sub Command1_Click()
Text1 = "Open:" & vbCrLf & _
ComdlgReplacement("Open", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)", Me)
End Sub
'command button for save dialog:
Private Sub Command2_Click()
Text1 = "Save:" & vbCrLf & _
ComdlgReplacement("Save", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)", Me)
End Sub
'You can see at all that comes next as a sort of a black box, it
just is in an mudule. All you need, is just the line from above, the
rest is done without further programming:
'Module modComDlgRep.mod
'Dieser Source stammt von http://www.soft-hummingbird.com
'und kann frei verwendet werden. Für eventuelle Schäden
'wird nicht gehaftet.
'This source code originates from http://www.soft-hummingbird.com
'it is as-is without any warranty and can be used freely
'In no event shall the author be held liable for any damages arising
from the use of this code
Option Explicit
'declaration of APIs
Private Declare Function GetOpenFileName
Lib "comdlg32.dll"
Alias "GetOpenFileNameA"
(pOpenfilename As OPENFILENAME)
As Long
Private Declare Function GetSaveFileName
Lib "comdlg32.dll"
Alias "GetSaveFileNameA"
(pOpenfilename As OPENFILENAME)
As Long
Private Declare Function PathIsDirectory
Lib "shlwapi.dll"
Alias "PathIsDirectoryA"
(ByVal pszPath As String)
As Long
'declaration of type
Private Type OPENFILENAME
lStructSize As Long
hwndOwner As Long
hInstance As Long
lpstrFilter As String
lpstrCustomFilter As String
nMaxCustFilter As Long
nFilterIndex As Long
lpstrFile As String
nMaxFile As Long
lpstrFileTitle As String
nMaxFileTitle As Long
lpstrInitialDir As String
lpstrTitle As String
flags As Long
nFileOffset As Integer
nFileExtension As Integer
lpstrDefExt As String
lCustData As Long
lpfnHook As Long
lpTemplateName As String
End Type
'to see all the APIs and declarations you could use go to:
'C:\Program Files\Microsoft Visual Studio\Common\Tools\Winapi
'there is a Viewer called "APILOAD.EXE"
'and text files with all the declarations you need ("WIN32API.TXT")
'Example:
'txtFile = "Open:" & vbCrLf & _
ComdlgReplacement("Open", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)",
Me)
'SaveOpen = "Save" or "Open"
'InitDir = "C:\Initial Directory\"
'sFilter = "Filename (*.*)", e.g. "All Files (*.*)" or "Text Files
(*.txt)"
'sFilter = "All Files (*.*)| Text Files (*.txt)| Batch Files
(*.bat)" -> multiple separated by "|"
'ComdlgReplacement = "" if cancel pressed
'ComdlgReplacement = "File" if file is selected
Public Function ComdlgReplacement(ByVal SaveOpen
As String, _
ByVal sInitDir As String, _
ByVal sFilter As String, _
ByVal CallingForm As Form)
As String
Dim OpenSaveFile As OPENFILENAME
Dim lReturn As Long
Dim sReturnFile As String
Dim sFilt As String
Dim h As String
Dim F() As String, FF()
As String
Dim i As Integer
If IsFolder(sInitDir)
Then
OpenSaveFile.lpstrInitialDir = sInitDir
Else
OpenSaveFile.lpstrInitialDir = "C:\" 'or something like: App.Path
End If
'Filter:
sFilter = Replace(sFilter, "| ", "|")
'"All Files (*.*) | Text Files
(*.txt)" -> "All Files (*.*) |Text Files (*.txt)" and now easy to
split:
sFilter = Replace(sFilter, " |", "|")
'"All Files (*.*) |Text Files
(*.txt)" -> "All Files (*.*)|Text Files (*.txt)" and now easy to
split:
F = Split(sFilter, "|")
'e.g.
'F(0) = "All Files (*.*)"
'F(1) = "Text Files (*.txt)"
'F(2) = "Batch Files (*.bat)"
For i = 0 To UBound(F)
h = F(i) 'F(i) = "All Files (*.*)"
h = Replace(h, " (", "(")
'h = "All Files (*.*)" -> "All Files(*.*)"
h = Replace(h, ")", "")
'h = "All Files (*.*)2 -> "All Files(*.*" -> now easy
to split
FF = Split(h, "(")
'FF(0) = "All Files": FF(1) ="*.*"
If UBound(FF) = 1 Then
sFilt = sFilt & F(i) & Chr(0) & FF(1) &
Chr(0)
End If
Next
OpenSaveFile.lpstrFilter = sFilt
OpenSaveFile.nFilterIndex = 1
OpenSaveFile.lStructSize = Len(OpenSaveFile)
OpenSaveFile.hwndOwner = CallingForm.hWnd
OpenSaveFile.hInstance = App.hInstance
OpenSaveFile.lpstrFile = String(257, 0)
OpenSaveFile.nMaxFile = Len(OpenSaveFile.lpstrFile) - 1
OpenSaveFile.lpstrFileTitle = OpenSaveFile.lpstrFile
OpenSaveFile.nMaxFileTitle = OpenSaveFile.nMaxFile
OpenSaveFile.flags = 0
If LCase(SaveOpen) = "save"
Then
OpenSaveFile.lpstrTitle = "Save"
lReturn = GetSaveFileName(OpenSaveFile)
ElseIf LCase(SaveOpen) = "open"
Then
OpenSaveFile.lpstrTitle = "Open"
lReturn = GetOpenFileName(OpenSaveFile)
End If
If lReturn = 0 Then
sReturnFile = ""
Else
sReturnFile = Trim(OpenSaveFile.lpstrFile)
End If
ComdlgReplacement = sReturnFile
End Function
Public Function IsFolder(ByVal Path
As String) As Boolean
IsFolder = CBool(PathIsDirectory(Path))
End Function
|
And here is how it looks: without ocx file, just with the dll and API:
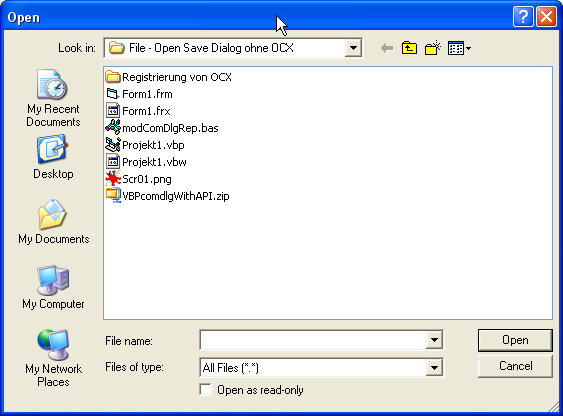
Download this source code embedded in an easy to use Visual Basic 6.0 project:
|
|