|
Programme
File Backupper
Digitize Plot To Data
Ready Replace
Scrabbler
Tutorials
Comsol 4.2 Tutorials
Gwyddion Tutorials
VB 6.0 Tutorials
Tutorials: sonst
|
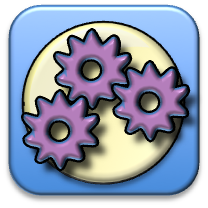 |
Tutorials |
VB 6.0 Tutorial: Common Dialog
ohne OCX 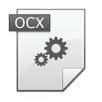
Dieses Tutorial behandelt wie man in Visual Basic 6.0 den Öffnen/
Speichern Dialog, welcher im comdlg32.ocx Modul ist
benutzt, ohne diese ocx Datei auf dem Computer haben zu müssen, ohne
diese Datei registrieren zu müssen und dementprechend keine
Administrator Rechte braucht.
Problem
Diese Fehlermeldung erscheint, wenn man ein Programm öffnen möchte,
welches diese ocx Datei voraussetzt/ verwendet und sie nicht auf dem
System installiert ist oder nicht registriert ist:
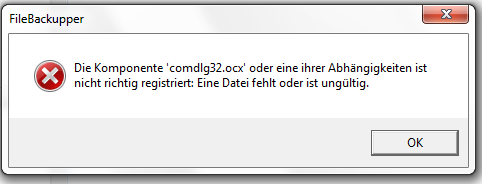
Die Komponente 'comdlg32.ocx' oder eine ihrer Abhängigkeiten ist nicht richtig registriert. Eine Datei fehlt oder ist ungültig.
Gewöhnlicherweise muss man dann diese ocx Datei in das C:\Windows\system32
Verzeichnis (in Windows XP) oder in das C:\Windows\SysWOW64\
Verzeichnis (in 64bit Windows Vista/ Windows 7) kopieren, um das
Programm öffnen zu können. Danach muss man diese Datei registrieren.
Dafür geht man auf Start -> Ausführen und tippt dort cmd ein, um in die
Komandozeilen Konsole zu gelangen. Dort tippt man folgenden Text oder
Sie führen die BAT Datei runter:
Windows 95, 98, oder Me:
C:\Windows\system\comdlg32.OCX
regsvr32 comdlg32.ocx
|
 |
In Windows NT, 2000:
copy comdlg32.ocx
C:\WINNT\system32\comdlg32.OCX
regsvr32 comdlg32.ocxpan> |
 |
In Windows XP:
copy comdlg32.ocx
C:\Windows\system32\comdlg32.OCX
regsvr32 comdlg32.ocx |
 |
oder in Windows 7:
copy comdlg32.ocx C:\Windows\SysWOW64\comdlg32.ocx regsvr32 C:\Windows\SysWOW64\comdlg32.ocx |
 |
Klicken Sie auf das
Icon, um eine BAT Datei runterzuladen, welche diese Befehle beinhaltet.
Man benötigt jedoch Administratorrechte, wenn man in die System Ordner
schrieben möchte (z.B. in C:\Windows\SysWOW64\ in Windows 7)
oder die ocx Datei registrieren möchte. Wenn man diese Rechte nciht hat,
erhält man eine Fehlermeldung mit folgender Nummer: 0x8002801c
Lösung
Im folgenden VB 6.0 Projekt lernen Sie, wie man direkt die comdlg32.dll
Datei (welche auf Window 7 bereits installiert ist) verwenden kann und
mit APIs den Öffnen/ Speichern Dialog benutzen kann. Dadurch braucht man
die ocx Datei nicht mit seinem Programm mitzuliefern und braucht sie
auch nicht zu registrieren. Administratorrechte braucht man dann auch
nicht, um das Programm zum laufen zu kriegen.
Keywords:
comdlg32.ocx common dialog Ersatz Umgehen
common dialog mit Win32 API
(5 kB)
Hier können Sie das Projekt runterladen, welches genau erklärt, wie das
funktioniert.
Und das ist der Quelltext:
Option Explicit
'command button for open dialog:
Private Sub Command1_Click()
Text1 = "Open:" & vbCrLf & _
ComdlgReplacement("Open", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)", Me)
End Sub
'command button for save dialog:
Private Sub Command2_Click()
Text1 = "Save:" & vbCrLf & _
ComdlgReplacement("Save", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)", Me)
End Sub
'You can see at all that comes next as a sort of a black box, it
just is in an module. All you need, is just the line from above, the
rest is done without further programming:
'Module modComDlgRep.mod
'Dieser Source stammt von http://www.soft-hummingbird.com
'und kann frei verwendet werden. Für eventuelle Schäden
'wird nicht gehaftet.
'This source code originates from http://www.soft-hummingbird.com
'it is as-is without any warranty and can be used freely
'In no event shall the author be held liable for any damages arising
from the use of this code
Option Explicit
'declaration of APIs
Private Declare Function GetOpenFileName
Lib "comdlg32.dll"
Alias "GetOpenFileNameA"
(pOpenfilename As OPENFILENAME)
As Long
Private Declare Function GetSaveFileName
Lib "comdlg32.dll"
Alias "GetSaveFileNameA"
(pOpenfilename As OPENFILENAME)
As Long
Private Declare Function PathIsDirectory
Lib "shlwapi.dll"
Alias "PathIsDirectoryA"
(ByVal pszPath As String)
As Long
'declaration of type
Private Type OPENFILENAME
lStructSize As Long
hwndOwner As Long
hInstance As Long
lpstrFilter As String
lpstrCustomFilter As String
nMaxCustFilter As Long
nFilterIndex As Long
lpstrFile As String
nMaxFile As Long
lpstrFileTitle As String
nMaxFileTitle As Long
lpstrInitialDir As String
lpstrTitle As String
flags As Long
nFileOffset As Integer
nFileExtension As Integer
lpstrDefExt As String
lCustData As Long
lpfnHook As Long
lpTemplateName As String
End Type
'to see all the APIs and declarations you could use go to:
'C:\Program Files\Microsoft Visual Studio\Common\Tools\Winapi
'there is a Viewer called "APILOAD.EXE"
'and text files with all the declarations you need ("WIN32API.TXT")
'Example:
'txtFile = "Open:" & vbCrLf & _
ComdlgReplacement("Open", App.Path, _
"All Files (*.*) | Text Files (*.txt) | VB Project Files (*.vbp)",
Me)
'SaveOpen = "Save" or "Open"
'InitDir = "C:\Initial Directory\"
'sFilter = "Filename (*.*)", e.g. "All Files (*.*)" or "Text Files
(*.txt)"
'sFilter = "All Files (*.*)| Text Files (*.txt)| Batch Files
(*.bat)" -> multiple separated by "|"
'ComdlgReplacement = "" if cancel pressed
'ComdlgReplacement = "File" if file is selected
Public Function ComdlgReplacement(ByVal SaveOpen
As String, _
ByVal sInitDir As String, _
ByVal sFilter As String, _
ByVal CallingForm As Form)
As String
Dim OpenSaveFile As OPENFILENAME
Dim lReturn As Long
Dim sReturnFile As String
Dim sFilt As String
Dim h As String
Dim F() As String, FF()
As String
Dim i As Integer
If IsFolder(sInitDir)
Then
OpenSaveFile.lpstrInitialDir = sInitDir
Else
OpenSaveFile.lpstrInitialDir = "C:\" 'or something like: App.Path
End If
'Filter:
sFilter = Replace(sFilter, "| ", "|")
'"All Files (*.*) | Text Files
(*.txt)" -> "All Files (*.*) |Text Files (*.txt)" and now easy to
split:
sFilter = Replace(sFilter, " |", "|")
'"All Files (*.*) |Text Files
(*.txt)" -> "All Files (*.*)|Text Files (*.txt)" and now easy to
split:
F = Split(sFilter, "|")
'e.g.
'F(0) = "All Files (*.*)"
'F(1) = "Text Files (*.txt)"
'F(2) = "Batch Files (*.bat)"
For i = 0 To UBound(F)
h = F(i) 'F(i) = "All Files (*.*)"
h = Replace(h, " (", "(")
'h = "All Files (*.*)" -> "All Files(*.*)"
h = Replace(h, ")", "")
'h = "All Files (*.*)2 -> "All Files(*.*" -> now easy
to split
FF = Split(h, "(")
'FF(0) = "All Files": FF(1) ="*.*"
If UBound(FF) = 1 Then
sFilt = sFilt & F(i) & Chr(0) & FF(1) &
Chr(0)
End If
Next
OpenSaveFile.lpstrFilter = sFilt
OpenSaveFile.nFilterIndex = 1
OpenSaveFile.lStructSize = Len(OpenSaveFile)
OpenSaveFile.hwndOwner = CallingForm.hWnd
OpenSaveFile.hInstance = App.hInstance
OpenSaveFile.lpstrFile = String(257, 0)
OpenSaveFile.nMaxFile = Len(OpenSaveFile.lpstrFile) - 1
OpenSaveFile.lpstrFileTitle = OpenSaveFile.lpstrFile
OpenSaveFile.nMaxFileTitle = OpenSaveFile.nMaxFile
OpenSaveFile.flags = 0
If LCase(SaveOpen) = "save"
Then
OpenSaveFile.lpstrTitle = "Save"
lReturn = GetSaveFileName(OpenSaveFile)
ElseIf LCase(SaveOpen) = "open"
Then
OpenSaveFile.lpstrTitle = "Open"
lReturn = GetOpenFileName(OpenSaveFile)
End If
If lReturn = 0 Then
sReturnFile = ""
Else
sReturnFile = Trim(OpenSaveFile.lpstrFile)
End If
ComdlgReplacement = sReturnFile
End Function
Public Function IsFolder(ByVal Path
As String) As Boolean
IsFolder = CBool(PathIsDirectory(Path))
End Function
|
So sieht dieses Projekt aus, welches keine eingebundene ocx Datei hat,
sondern die dll benutzt:
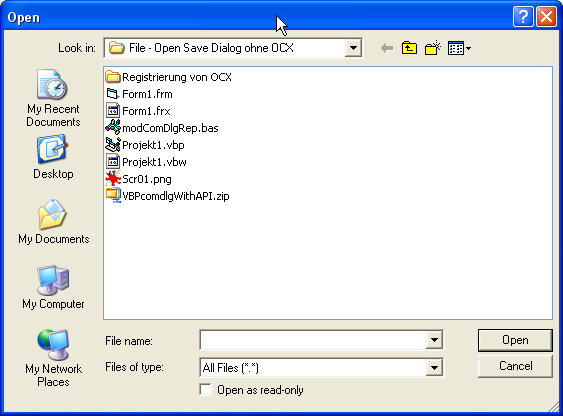
Zum runterladen für VB 6.0:
(5 kB)
|
|